Share this article

How Vanta's engineering team improved productivity with esbuild
Accelerating security solutions for small businesses Tagore offers strategic services to small businesses. | A partnership that can scale Tagore prioritized finding a managed compliance partner with an established product, dedicated support team, and rapid release rate. | Standing out from competitors Tagore's partnership with Vanta enhances its strategic focus and deepens client value, creating differentiation in a competitive market. |
As of early 2022, Vanta’s backend consists of Typescript services and lambdas, and our frontend is a React and Typescript-based single-page application. We host all this code in a monorepo. Over time, we experienced build slowdowns due to our organically growing codebase.
Ultimately, we decided to use esbuild to ensure developer edit/refresh cycles stay smooth. In this article, we’ll explore why we made this decision, how we implemented esbuild, and the results that followed.
The challenge
Our codebase has always been structured as a tree of packages. The leaf directories are services that include a common directory and are bundled up by a Dockerfile. For development, we have a docker compose setup that spins up all services, and essentially runs tsc --watch for each service.
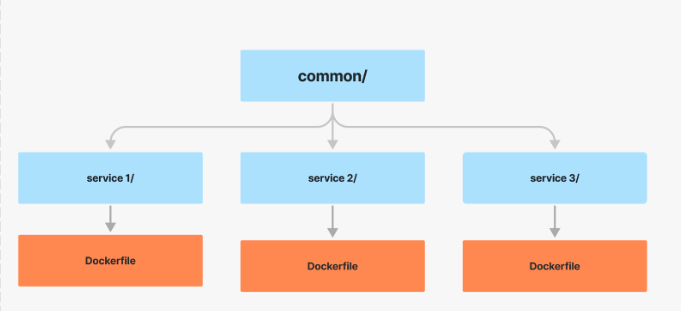
Back in the early days, we leveraged symlinks to manage the dependency tree, which we replaced with Typescript Project References to simplify our setup and accelerate builds.
We experienced reasonable performance with this setup for a few years until tsc --watch incremental builds were slowing down. It was taking tens of seconds to re-compile our backend for even the most minimal changes. Our first recourse was to understand the problem using Typescript’s great performance debugging guide. We discovered a few type-heavy packages, like our very own ts-json-validator, and certain usage patterns of mongoose were leading to severe slowdowns. Through a series of casts and manually provided types, we relieved some burden from Typescript's automatic inference and managed to cut build times by >50%. Even then, with a growing engineering team and codebase, we knew that this wouldn’t be a long-term solution, and re-compilation times steadily crept back up.
After another round of Typescript profiling, we noticed an interesting comment on HackerNews that talked about replacing the Typescript compiler with esbuild. After a quick manual prototype, we found that esbuild could re-compile one of our smaller services in under a second, so we decided to investigate whether we could replace all our transpilation with esbuild.
What is esbuild?
esbuild is a fast Javascript bundler written in Go that focuses on correctness and performance. Bundlers traditionally take existing source code and “bundle” it up into a smaller output file so that it can be served faster over the internet, but over the years, bundlers have taken on additional responsibilities, like transpiling from Typescript to Javascript. All we really needed for backend code was that transpilation step, along with a few niceties like source maps for better stack traces in our observability tools.
The strategy
EsModuleInterop
Our biggest roadblock to enabling esbuild globally in our codebase was that we didn’t have the Typescript option esModuleInterop enabled.
Understanding the module ecosystem
Modern programming languages have a module system so developers can separate code with reasonable boundaries into a self-contained package or module and import that code somewhere else. Javascript, famously written in ten days, did not start off with a module system. Over time, one working group came up with commonjs for modules in Node. Sometime after, the community came up with a different module system—ES Modules, or ESM, with dedicated Javascript syntax for imports. The Javascript community is standardizing ES Modules since they’re the in-built system, and have slightly better and stricter semantics. Here’s a good blog on the intricacies and nuances of the two systems.
Difference in syntax

How Typescript fits in
Typescript added import statements before Javascript natively supported import. And Typescript transpiled import * as statements into CommonJS require statements:


This breaks compatibility with the new ESM spec, as CommonJS require has looser semantics than ES6 import * as. For example, require is allowed to return a non-object, whereas import * as is required to return a plain object. This behavior goes against one of their stated design goals to align with ECMAScript proposals.
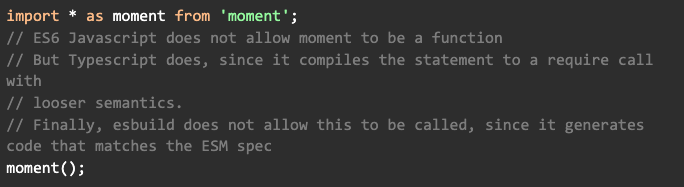
Since Typescript’s generated code didn’t conform to the spec, they released an option for interoperability called esModuleInterop. They’ve enabled it by default for new projects, and highly recommend that it’s enabled for all projects going forward. esbuild does not need to break the spec, so its generated code has different semantics than Typescript generated code with esModuleInterop: false. For example, esbuild generates code that assumes imported modules are not callable and are read-only. This is such a common issue that there’s documentation about it, and the recommended path forward is to enable esModuleInterop for codebases to use esbuild.
We needed to take on two kinds of tasks to correctly enable this option: re-do our imports and clean up mocking code.
Re-doing imports
esModuleInterop automatically enables allowSyntheticDefaultImports, so we migrated many of our imports. This wasn’t strictly required, but it was the cleaner end state with this option enabled.
Example:

to

Unfortunately, this led to a few breakages that weren’t caught at compile-time, since this only retained the same behavior for packages that didn’t have pre-existing default exports. We discovered most bugs here through testing and deployment in our staging environment.
Re-doing mocks
We use sinon for our mocks in tests. An example:
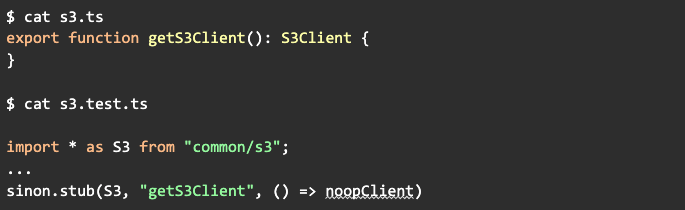
This would no-longer work with esModuleInterop because ES modules are not directly assignable. We were essentially modifying code that was imported, which is a code-smell. We decided to remove mocks where it was easy, and export objects that sinon could mutate when it wasn’t, rather than trying to redo the import itself. This was another common issue with a lot of discussion in the community.

The changes here were pretty mechanical as soon as we got a handle on the problem, and it took one PR for each of the handful of modules affected.
These migrations were the bulk of the work required before we could use esbuild.
Build script
With our migration to esModuleInterop complete, we wrote a bash script that essentially set up nodemon to watch our Typescript files, and re-ran esbuild on changes.
A large issue worth noting here is that esbuild is explicitly not a Typescript compiler replacement, so it doesn’t grok project references. Fortunately, since it’s so fast, we rebuild all running services in parallel when there are any code changes to our common directories, and that’s worked out fine in practice. Another issue is that esbuild doesn’t recognize JSON entry-points, so it doesn’t copy over JSON files into the output directory which can be imported with resolveJsonModule: true in Typescript. So we just copy those over manually through a find and cp.
The rollout
First, since esbuild is not a Typescript compiler, we made it clear to developers that their code might rebuild successfully through esbuild, even if compilation really should have failed due to type errors. Instead, they could use editors to find most compilation errors and run the Typescript compiler on watch mode manually while doing large refactors to catch compilation errors. We also run the Typescript compiler in CI to make sure that no compilation errors get through. Developers seemed to prefer this trade-off.
Second, our team strongly believes that our systems are as consistent as possible in development and production. Therefore, if developers were interacting with esbuild-built code locally, we should ship esbuild-built code to production. To roll this out, we first enabled esbuild for local development behind an opt-in flag. Next, we pushed esbuild-built code to our staging environment, and finally to production. After almost a year in production, we haven’t noticed any issues with the exception of missing source maps which we fixed by tweaking a few flags.
The results
Overall, we’ve been satisfied with our esbuild experience. Looking back, we should have looked into swc a little more, especially since we used Parcel for our front-end code and Parcel 2 uses swc behind the scenes. But it seems easy to switch from one to the other since the bulk of the work was not strictly related to esbuild.
Most developers aren’t aware that we use esbuild behind the scenes, and we count that as a success. On the other hand, we’ve run into more issues around the Typescript server in code editors, mainly around OOMs due to pathological third-party dependencies and organic codebase and dependency growth, so we’ll continue investing on that front.
Credits go to Evan Wallace, the author behind esbuild, for helping push the Javascript ecosystem towards better, faster tooling. If you’re interested in solving tricky problems to make the internet a more secure place, we’re hiring! Check out our open roles here.
Learn more about engineering at Vanta
How Vanta supports a distributed engineering team
3 GraphQL pitfalls and steps to avoid them
9 security tips for startups (and how coding plays a part)




Determine if you need to comply with GDPR
Not all organizations are legally required to comply with the GDPR, so it’s important to know how this law applies to your organization. Consider the following:
Do you sell goods or services in the EU or UK?
Do you sell goods or services to EU businesses, consumers, or both?
Do you have employees in the EU or UK?
Do persons from the EU or UK visit your website?
Do you monitor the behavior of persons within the EU?
Document the personal data you process
Because GDPR hinges on the data you collect from consumers and what your business does with that data, you’ll need to get a complete picture of the personal data you’re collecting, processing, or otherwise interacting with. Follow these items to scope out your data practices:
Identify and document every system (i.e. database, application, or vendor) that stores or processes EU- or UK-based personally identifiable information (PII).
Document the retention periods for PII in each system.
Determine whether you collect, store, or process “special categories” of data, including:
Determine whether your documentation meets the GDPR requirements for Records of Processing Activities, that include information on:
Determine whether your documentation includes the following information about processing activities carried out by vendors on your behalf:
Determine your legal grounds for processing data
GDPR establishes conditions that must be met before you can legally collect or process personal data. Make sure your organization is meeting the conditions listed below:
For each category of data and system/application, determine the lawful basis for processing based on one of the following conditions:
Review and update current customer and vendor contracts
For your organization to be fully GDPR compliant, the vendors you use must also maintain the privacy rights of your users’ and those rights should be reflected in your contracts with customers:
Review all customer and in-scope vendor contracts to determine that they have appropriate contract language (i.e. Data Protection Addendums with Standard Contractual Clauses).
Determine if you need a Data Protection Impact Assessment
A Data Protection Impact Assessment (DPIA) is an assessment to determine what risks may arise from your data processing and steps to take to minimize them. Not all organizations need a DPIA, the following items will help you determine if you do:
Identify if your data processing is likely to create high risk to the rights and freedoms of natural persons. Considering if your processing involves any of the following:
Clearly communicate privacy and marketing consent practices
A fundamental element of GDPR compliance is informing consumers of their data privacy rights and requesting consent to collect or process their data. Ensure your website features the following:
A public-facing privacy policy which covers the use of all your products, services, and websites.
Notice to the data subject that include the essential details listed in GDPR Article 13.
Have a clear process for persons to change or withdraw consent.
Update internal privacy policies
Ensure that you have privacy policies that are up to the standards of GDPR:
Update internal privacy notices for EU employees.
Have an employee privacy policy that governs the collection and use of EU and UK employee data.
Determine if you need a data protection officer (DPO) based on one of the following conditions:
Review compliance measures for external data transfers
Under GDPR, you’re responsible for protecting the data that you collect and if that data is transferred. Make your transfer process compliant by following these steps:
If you transfer, store, or process data outside the EU or UK, identify your legal basis for the data transfer. This is most likely covered by the standard contractual clauses.
Perform and document a transfer impact assessment (TIA).
Confirm you comply with additional data subject rights
Ensure you’re complying with the following data subject rights by considering the following questions:
Do you have a process for timely responding to requests for information, modifications, or deletion of PII?
Can you provide the subject information in a concise, transparent, intelligible, and easily accessible form, using clear and plain language?
Do you have a process for correcting or deleting data when requested?
Do you have an internal policy regarding a Compelled Disclosure from Law Enforcement?
Determine if you need an EU-based representative
Depending on how and where your organization is based, you may need a representative for your organization within the European Union. Take these steps to determine if this is necessary:
Determine whether an EU representative is needed. You may not need an EU-rep if the following conditions apply to your organization:
If the above conditions do not apply to you, appoint an EU-based representative.
Identify a lead data protection authority (DPA) if needed
GDPR compliance is supervised by the government of whatever EU member-state you’re operating in. If you’re operating in multiple member-states, you may need to determine who your lead data protection authority is:
Determine if you operate in more than one EU state.
If so, designate the supervisory authority of the main establishment to act as your DPA.
Implement employee training
Every employee in your organization provides a window for hackers to gain access to your systems and data. This is why it's important to train your employees on how to prevent security breaches and maintain data privacy:
Provide appropriate security awareness and privacy training to your staff.
Integrate data breach response requirements
GDPR requires you to create a plan for notifying users and minimizing the impact of a data breach. Examine your data breach response plan, by doing the following:
Create and implement an incident response plan which includes procedures for reporting a breach to EU and UK data subjects as well as appropriate data authorities.
Establish breach reporting policies that comply with all prescribed timelines and include all recipients (i.e. authorities, controllers, and data subjects).
Implement appropriate security measures
Have you implemented encryption of PII at rest and in transit?
Have you implemented pseudonymization?
Have you implemented appropriate physical security controls?
Have you implemented information security policies and procedures?
Can you access EU or UK PII data in the clear?
Do your technical and organizational measures ensure that, by default, only personal data that are necessary for each specific purpose of the processing are processed?
Streamline GDPR compliance with automation
GDPR compliance is an ongoing project that requires consistent upkeep with your system, vendors, and other factors that could break your compliance. Automation can help you stay on top of your ongoing GDPR compliance. The following items can help you streamline and organize your continuous compliance:
Explore tools for automating security and compliance.
Transform manual data collection and observation processes via continuous monitoring.
Download this checklist for easy reference
GDPR compliance FAQs
In this section, we’ve answered some of the most common questions about GDPR compliance:
What are the seven GDPR requirements?
The requirements for GDPR compliance are based on a set of seven key principles:
- Lawfulness, fairness, and transparency
- Purpose limitation
- Data minimization
- Accuracy
- Storage limitations
- Integrity and confidentiality
- Accountability
These are the seven requirements you must uphold to be GDPR compliant.
Is GDPR compliance required in the US?
GDPR compliance is mandatory for some US companies. GDPR compliance is not based on where your organization is located but whose data you collect, store, or process. Regardless of where your organization is based, you must comply with GDPR if you are collecting or processing data from EU residents.
What are the four key components of GDPR?
The four components of GDPR include:
- Data protection principles
- Rights of data subjects
- Legal bases for data processing
- Responsibilities and obligations of data controllers and processors
Safeguard your business with GDPR compliance
If your organization collects data from EU residents, GDPR compliance is mandatory for you. It’s important to follow the steps listed above to protect your business from heavy fines and to respect the data privacy rights of consumers.
Vanta provides compliance automation tools and continuous monitoring capabilities that can help you get and stay GDPR compliant. Learn more about getting GDPR compliance with Vanta.
Pre-work for your SOC 2 compliance
Choose the right type of SOC 2 report:
A SOC 2 Type 1 report assesses how your organization aligns with the security controls and policies outlined in SOC 2
A SOC 2 Type 2 report has all the components of a Type 1 report with the addition of testing your controls over a period of time
The correct report will depend on the requirements or requests of the client or partner that has requested a SOC 2 report
from you
Determine the framework for your SOC 2 report. Of the five Trust Service Criteria in SOC 2, every organization needs to comply with the first criteria (security), but you only need to assess and document the other criteria that apply. Determining your framework involves deciding which Trust Service Criteria and controls are applicable to your business using our Trust Service Criteria Guide.
Estimate the resources you expect to need. This will vary depending on how closely you already align with SOC 2 security controls, but it can include several costs such as:
Compliance software
Engineers and potentially consultants
Security tools, such as access control systems
Administrative resources to draft security policies
Auditing for your compliance certification
Obtain buy in from your organization leadership to provide the resources your SOC 2 compliance will need.
Work toward SOC 2 compliance
Begin with an initial assessment of your system using compliance automation software to determine which necessary controls and practices you have already implemented and which you still need to put in place.
Review your Vanta report to determine any controls and protocols within the “Security” Trust Service Criteria that you do not yet meet and implement these one by one. These are multi-tiered controls across several categories of security, including:
CC1: Control Environment
CC2: Communication and Information
CC3: Risk Assessment
CC4: Monitoring Activities
CC5: Control Activities
CC6: Logical and Physical Access Controls
CC7: System Operations
CC8: Change Management
CC9: Risk Mitigation
Using Vanta’s initial assessment report as a to-do list, address each of the applicable controls in the other Trust Services Criteria that you identified in your initial framework, but that you have not yet implemented.
Using Vanta’s initial assessment report, draft security policies and protocols that adhere to the standards outlined in SOC 2.
Vanta’s tool includes thorough and user-friendly templates to make this simpler and save time for your team.
Run Vanta’s automated compliance software again to determine if you have met all the necessary criteria and controls for your SOC 2 report and to document your compliance with these controls.
Complete a SOC 2 report audit
Select and hire an auditor affiliated with the American Institute of Certified Public Accountants (AICPA), the organization that developed and supports SOC 2.
Complete a readiness assessment with this auditor to determine if you have met the minimum standards to undergo a full audit.
If your readiness assessment indicates that there are SOC 2 controls you need to address before your audit, complete these requirements. However, if you have automated compliance software to guide your preparations and your SOC 2 compliance, this is unlikely.
Undergo a full audit with your SOC 2 report auditor. This may involve weeks or longer of working with your auditor to provide the documentation they need. Vanta simplifies your audit, however, by compiling your compliance evidence and documentation into one platform your auditor can access directly.
When you pass your audit, the auditor will present you with your SOC 2 report to document and verify your compliance.
Maintain your SOC 2 compliance annually
Establish a system or protocol to regularly monitor your SOC 2 compliance and identify any breaches of your compliance, as this can happen with system updates and changes.
Promptly address any gaps in your compliance that arise, rather than waiting until your next audit.
Undergo a SOC 2 re-certification audit each year with your chosen SOC 2 auditor to renew your certification.
Download this checklist for easy reference
Prioritizing Your Security and Opening Doors with SOC 2 Compliance
Information security is a vital priority for any business today from an ethical standpoint and from a business standpoint. Not only could a data breach jeopardize your revenue but many of your future clients and partners may require a SOC 2 report before they consider your organization. Achieving and maintaining your SOC 2 compliance can open countless doors, and you can simplify the process with the help of the checklist above and Vanta s compliance automation software. Request a demo today to learn more about how we can help you protect and grow your organization.
Pre-work for your ISO 42001 compliance
Understand ISO 42001 requirements
Decide on what is the scope of the AIMS
Familiarize yourself with key AI concepts, principles, and lifecycle based on ISO frameworks
Determine if you are a provider, developer, or user of AI systems
Perform initial gap analysis
Using Vanta, asses your in-scope ISO 42001 controls
Identify areas of requirement, development, or adjustment
Secure top management support
Present a business case highlighting the benefits of ISO 42001 certification
Define roles and responsibilities for top management in AIMS implementation
Involve various department heads in the analysis to ensure comprehensive coverage
Work for your ISO 42001 compliance
Appoint a Project Manager
Designate an owner for the ISO 42001 implementation project
Develop a project plan
Outline steps, timelines, and resources needed for AIMS implementation
Integrate the AIMS implementation project within existing organizational processes
Establish the AIMS framework
Define the scope and objectives of the AIMS within the organization
Develop and document AI policies and risk management processes
Based on gap analysis, implement necessary controls for AIMS
Ensure integration of AIMS with other management systems and requirements
Create an AIMS statement of applicability (SOA)
Promote competence and awareness
Conduct training for stakeholders on AI concepts and ISO 42001 requirements
Raise awareness about the importance and benefits of AIMS
Implement AIMS controls
Create an AI policy
Define the process for reporting concerns about AI systems
Identify, document, and manage resources for AI systems
Ensure tooling and computing resources for AI systems are adequately documented
Conduct an AI system impact assessment exercise
Ensure that objectives are documented for the design and development of AI systems
Create a process for responsible design and development of AI systems
Ensure that AI system deployment, operation, and monitoring are documented and executed according to your AIMS
Define and implement data management processes for AI systems
Assess and document the quality of data for AI systems
Ensure that system documentation and information for users is provided and accessible
Document and follow the processes for the responsible use of AI systems
Clearly allocate and document responsibilities with third parties
Conduct internal audits
Regularly assess compliance with ISO 42001 and the effectiveness of AIMS
Management review
Review AIMS performance and compliance with top management
Address any non conformities and areas for improvement
Prepare for your external audit
Work with A-LIGN as your ISO 42001 certification body
Engage A-LIGN, a leading ISO certification body, to conduct your audit
Prepare documentation
Ensure all AIMS documentation is up to date and accessible
Pre-audit meeting
Prepare a list of questions and clarifications regarding the audit process
Initial sales process
Discuss the scope of the audit in detail to ensure full preparedness
Conduct a pre-certification audit (optional)
Consider a pre-certification audit to identify any remaining gaps
The ISO 42001 audit
Engage in the certification audit
Collaborate with A-LIGN auditors, providing necessary information and access
Designate a team member as the point of contact for auditors to streamline communication
Organize walkthroughs to discuss your AIMS processes and procedures, including facilities (if applicable)
Address audit findings
Plan for immediate, short-term, and long-term corrective actions based on the audit report
Celebrate the audit success with your team and publicly promote your certification
Continuous improvement
Establish a continuous improvement team to oversee progress post-certification
Continuously improve the AIMS, leveraging lessons learned and feedback
Integrate ISO 42001 compliance metrics into regular management reviews
Keys to success
Leverage Vanta s readiness capabilities and A-LIGN s expertise for an efficient and high-quality audit experience from
readiness to report
Incorporate AIMS within the business strategy and daily operations
Apply continuous improvement to enhance AIMS over time
Avoid integrating new technologies during the initial AIMS implementation
Engage interested parties and maintain their support throughout
Highlight the completion of the audit to demonstrate trust with customers, partners, and other key stakeholders
Download this checklist for easy reference
Demonstrating secure AI practices with ISO 42001
The rapid adoption of AI has driven innovation and opportunities for growth — and with it, new risks for the companies that manage the data that power these technologies. These companies have not had a way to demonstrate trust to their customers and show that they are deploying AI securely and safely. Achieving ISO 42001 compliance helps to demonstrate this trust through a third-party verifiable way and opens the doors to time-savings, more deals, and expedited sales processes. The above checklist simplifies the process of becoming ISO 42001 compliant by leveraging the power of Vanta's continuous compliance software. Request a demo today to learn more about how Vanta can help you streamline the path to ISO 42001.
Develop a roadmap for your ISMS implementation and ISO 27001 certification
Implement Plan, Do, Check, Act (PDCA) process to recognize challenges and identify gaps for remediation
Consider the costs of ISO 27001 certification relative to your organization’s size and number of employees.
Use project planning tools like project management software, Gantt charts, or Kanban boards.
Define the scope of work from planning to completion.
Determine the scope of your organization’s ISMS
Decide which business areas are covered by your ISMS and which ones are out of scope
Consider additional security controls for processes that are required to pass ISMS-protected information across the trust boundary.
Communicate the scope of your ISMS to stakeholders.
Establish an ISMS team and assign roles
Select engineers and technical staff with experience in information security to construct and implement the security controls needed for ISO 27001.
Build a governance team with management oversight.
Incorporate key members of top management (senior leadership and executive management) and assign responsibility for strategy and resource allocation.
If you have a large team, consider assigning a dedicated project manager to track progress and expedite implementation.
Align the team on the following:
The planning steps you’ve already taken
The scope of the ISMS
Which team members are responsible for which aspects of the project
Conduct an inventory of information assets
Consider all assets where information is stored, processed, and accessible
- Record information assets: data and people
- Record physical assets: laptops, servers, and physical building locations
- Record intangible assets: intellectual property, brand, and reputation
Assign to each asset a classification and owner responsible for ensuring the asset is appropriately inventoried, classified, protected, and handled
Meet with your team to discuss this inventory and ensure that everyone is aligned.
Perform a risk assessment
Establish and document a risk-management framework to ensure consistency
Identify scenarios in which information, systems, or services could be compromised
Determine likelihood or frequency with which these scenarios could occur
Evaluate potential impact of each scenario on confidentiality, integrity, or availability of information, systems, and services
Rank risk scenarios based on overall risk to the organization’s objectives
Develop a risk register
Record and manage your organization’s risks that you identified during your risk assessment.
Summarize each identified risk
Indicate the impact and likelihood of each risk.
Rank risk scenarios based on overall risk to the organization’s objectives.
Document a risk treatment plan
Design a response for each risk, known as a risk treatment.
Assign an owner to each identified risk and each risk mitigation activity.
Establish target timelines for completion of risk treatment activities.
Implement your risk mitigation treatment plan and track the progress of each task.
Complete the Statement of Applicability
Review the 93 controls listed in Annex A.
Select the controls that are relevant to the risks you identified in your risk assessment.
Complete the Statement of Applicability listing all Annex A controls, justifying inclusion or exclusion of each control in your ISMS implementation.
Implement ISMS policies, controls and continuously assess risk
Assign owners to each of the security controls to be implemented.
Figure out a way to track the progress and goals for each control.
Build a framework for establishing, implementing, maintaining, and continually improving the ISMS.
Include information or references to supporting documentation regarding:
- Information Security Objectives
- Leadership and Commitment
- Roles, Responsibilities, and Authorities
- Approach to Assessing and Treating Risk
- Control of Documented Information
- Communication
- Internal Audit
- Management Review
- Corrective Action and Continual Improvement
- Policy Violations
- All of the Annex A controls that you have selected
Establish employee training and awareness programs
Define expectations for personnel regarding their role in ISMS maintenance.
Train personnel on common threats facing your organization and how to respond.
Establish disciplinary or sanctions policies or processes for personnel found out of compliance with information security requirements.
Make security training part of the onboarding process for new employees.
Conduct regular training to ensure awareness of new policies and procedures.
Conduct regular management reviews
Plan reviews at least once per year. Consider a quarterly review cycle if your organization is large or if your infrastructure is changing frequently.
Ensure the ISMS and its objectives continue to be effective.
Verify that senior management stays informed.
Ensure risks or deficiencies can be promptly addressed.
Assemble ISO 27001 required documents
Review the ISO 27001 Required Documents and Records list.
Customize policy templates with organization-specific policies, process, and language.
Perform an ISO 27001 internal audit.
Examine each of the requirements from Annex A that you deemed applicable in your ISMS' Statement of Applicability and verify that you have each in place.
Assign in-house employees to conduct the internal audit, specifically employees who were not involved in the ISMS development and maintenance or hire an independent third party.
Share internal audit results, including nonconformities, with the ISMS team and senior management.
Address any issues your internal audit identified before proceeding with the external audit.
Verify compliance with the requirements from Annex A deemed applicable in your ISMS' Statement of Applicability.
Undergo external audit of ISMS to obtain ISO 27001 certification.
Select an independent ISO 27001 auditor.
Complete the Stage 1 Audit consisting of an extensive documentation review; obtain the auditor’s feedback regarding your readiness to move to the Stage 2 Audit.
Complete the Stage 2 Audit consisting of tests performed on the ISMS to ensure proper design, implementation, and ongoing functionality; evaluate fairness, suitability, and effective implementation and operation of controls.
Address any nonconformities.
Ensure that all requirements of the ISO 27001 standard are addressed.
Ensure your organization is following the processes that it has specified and documented.
Ensure your organization is upholding contractual requirements with third parties.
Address specific nonconformities identified by the ISO 27001 auditor.
Receive auditor’s formal validation following resolution of nonconformities.
Plan for subsequent ISO 27001 audits and surveillance audits.
Perform a full ISO 27001 audit once every three years
Prepare to perform surveillance audits in the second and third years of the Certification Cycle
Consider streamlining ISO 27001 certification with automation.
Transform manual data collection and observation processes into automated and continuous system monitoring
Identify and close any gaps in ISMS implementation in a timely manner
Learn more about achieving ISO 27001 certification with Vanta
Book an ISO 27001 demo with Vanta
Download this checklist for easy reference
Prioritizing your security and opening doors with ISO 27001 compliance
Information security is a vital priority for any business today from an ethical standpoint and from a business standpoint. Not only could a data breach jeopardize your revenue, but many of your future clients and partners may require an ISO 27001 report before they consider your organization. Achieving and maintaining your ISO 27001 compliance can open countless doors, and you can simplify the process with the help of the checklist above and Vanta’s compliance automation software.
Request a demo today to learn more about how we can help you protect and grow your organization.
Develop a roadmap for your ISMS implementation and ISO 27001 certification
Implement Plan, Do, Check, Act (PDCA) process to recognize challenges and identify gaps for remediation
Consider the costs of ISO 27001 certification relative to your organization’s size and number of employees.
Use project planning tools like project management software, Gantt charts, or Kanban boards.
Define the scope of work from planning to completion.
Determine the scope of your organization’s ISMS
Decide which business areas are covered by your ISMS and which ones are out of scope
Consider additional security controls for processes that are required to pass ISMS-protected information across the trust boundary.
Communicate the scope of your ISMS to stakeholders.
Establish an ISMS team and assign roles
Select engineers and technical staff with experience in information security to construct and implement the security controls needed for ISO 27001.
Build a governance team with management oversight.
Incorporate key members of top management (senior leadership and executive management) and assign responsibility for strategy and resource allocation.
If you have a large team, consider assigning a dedicated project manager to track progress and expedite implementation.
Align the team on the following:
The planning steps you’ve already taken
The scope of the ISMS
Which team members are responsible for which aspects of the project
Conduct an inventory of information assets
Consider all assets where information is stored, processed, and accessible
- Record information assets: data and people
- Record physical assets: laptops, servers, and physical building locations
- Record intangible assets: intellectual property, brand, and reputation
Assign to each asset a classification and owner responsible for ensuring the asset is appropriately inventoried, classified, protected, and handled
Meet with your team to discuss this inventory and ensure that everyone is aligned.
Perform a risk assessment
Establish and document a risk-management framework to ensure consistency
Identify scenarios in which information, systems, or services could be compromised
Determine likelihood or frequency with which these scenarios could occur
Evaluate potential impact of each scenario on confidentiality, integrity, or availability of information, systems, and services
Rank risk scenarios based on overall risk to the organization’s objectives
Develop a risk register
Record and manage your organization’s risks that you identified during your risk assessment.
Summarize each identified risk
Indicate the impact and likelihood of each risk.
Rank risk scenarios based on overall risk to the organization’s objectives.
Document a risk treatment plan
Design a response for each risk, known as a risk treatment.
Assign an owner to each identified risk and each risk mitigation activity.
Establish target timelines for completion of risk treatment activities.
Implement your risk mitigation treatment plan and track the progress of each task.
Complete the Statement of Applicability
Review the 93 controls listed in Annex A.
Select the controls that are relevant to the risks you identified in your risk assessment.
Complete the Statement of Applicability listing all Annex A controls, justifying inclusion or exclusion of each control in your ISMS implementation.
Implement ISMS policies, controls and continuously assess risk
Assign owners to each of the security controls to be implemented.
Figure out a way to track the progress and goals for each control.
Build a framework for establishing, implementing, maintaining, and continually improving the ISMS.
Include information or references to supporting documentation regarding:
- Information Security Objectives
- Leadership and Commitment
- Roles, Responsibilities, and Authorities
- Approach to Assessing and Treating Risk
- Control of Documented Information
- Communication
- Internal Audit
- Management Review
- Corrective Action and Continual Improvement
- Policy Violations
- All of the Annex A controls that you have selected
Establish employee training and awareness programs
Define expectations for personnel regarding their role in ISMS maintenance.
Train personnel on common threats facing your organization and how to respond.
Establish disciplinary or sanctions policies or processes for personnel found out of compliance with information security requirements.
Make security training part of the onboarding process for new employees.
Conduct regular training to ensure awareness of new policies and procedures.
Conduct regular management reviews
Plan reviews at least once per year. Consider a quarterly review cycle if your organization is large or if your infrastructure is changing frequently.
Ensure the ISMS and its objectives continue to be effective.
Verify that senior management stays informed.
Ensure risks or deficiencies can be promptly addressed.
Assemble ISO 27001 required documents
Review the ISO 27001 Required Documents and Records list.
Customize policy templates with organization-specific policies, process, and language.
Perform an ISO 27001 internal audit.
Examine each of the requirements from Annex A that you deemed applicable in your ISMS' Statement of Applicability and verify that you have each in place.
Assign in-house employees to conduct the internal audit, specifically employees who were not involved in the ISMS development and maintenance or hire an independent third party.
Share internal audit results, including nonconformities, with the ISMS team and senior management.
Address any issues your internal audit identified before proceeding with the external audit.
Verify compliance with the requirements from Annex A deemed applicable in your ISMS' Statement of Applicability.
Undergo external audit of ISMS to obtain ISO 27001 certification.
Select an independent ISO 27001 auditor.
Complete the Stage 1 Audit consisting of an extensive documentation review; obtain the auditor’s feedback regarding your readiness to move to the Stage 2 Audit.
Complete the Stage 2 Audit consisting of tests performed on the ISMS to ensure proper design, implementation, and ongoing functionality; evaluate fairness, suitability, and effective implementation and operation of controls.
Address any nonconformities.
Ensure that all requirements of the ISO 27001 standard are addressed.
Ensure your organization is following the processes that it has specified and documented.
Ensure your organization is upholding contractual requirements with third parties.
Address specific nonconformities identified by the ISO 27001 auditor.
Receive auditor’s formal validation following resolution of nonconformities.
Plan for subsequent ISO 27001 audits and surveillance audits.
Perform a full ISO 27001 audit once every three years
Prepare to perform surveillance audits in the second and third years of the Certification Cycle
Consider streamlining ISO 27001 certification with automation.
Transform manual data collection and observation processes into automated and continuous system monitoring
Identify and close any gaps in ISMS implementation in a timely manner
Learn more about achieving ISO 27001 certification with Vanta
Book an ISO 27001 demo with Vanta
Download this checklist for easy reference
Download NowPlan strategically when scheduling and timing your audit
Sometimes, the biggest roadblock to scheduling an audit is knowing where to start. Here are a few tips for taking your first big step in the audit process.
Check into your chosen firm’s availability. Small auditing firms tend to have more limited time and need more advance notice, while larger firms have more resources and, therefore, more availability. However, larger firms can cost more to hire.
Give yourself enough time before the audit. In many cases, audits don’t just check to see if you’ve done an activity once; they look for evidence of continuous controls. Plan to collect consistent evidence over at least three months to demonstrate this.
Choose a dedicated team or team member who will be available throughout the audit. Your auditor will need a go-to person to provide all the proper information and be available for any follow-up questions. Check calendars of others stakeholders involved with the audit controls to ensure they’ll be present when you schedule your audit on-site or live sessions.
Be aware of commitments and deadlines. You will likely sign a statement of work (SOW) when you schedule an audit. Your team is responsible for meeting all the deadlines outlined in this SOW. The auditor will need the correct information from your team in a timely manner to do their job.
Pro tip: Make sure your team understands the difference between the “audit window” or “review period” and the length of the audit itself. The “audit window” is the timeframe the auditors will use to gauge if you’ve been consistent with your controls. Typically, they require three months or more. This time period is different from the 1-4 weeks that the auditor will be inside your organization, conducting the audit itself.
Prepare your team in advance
Once you’ve scheduled your audit, it’s time to work with your team and assign tasks to the appropriate owners. An audit requires participation from several teams, from engineering to IT to sales. Here are a few ways to prepare these teams for audit-related activities:
Know which framework(s) you’re working towards and communicate the requirements to your team. If you’re going to work towards multiple frameworks simultaneously, identify overlaps so you don’t duplicate work. A tool like Vanta helps break down requirements and map them to action items.
Understand which evidence each team needs to provide. Next, communicate requirements and deadlines to each department. It’s a good idea to use a central hub that can show all preparation activities and who is responsible for each one.
Inform your team why this is an important activity so they understand that the time and commitment are worthwhile for them. Connect it to outcomes and KPIs that matter to their teams whenever possible. Audits ultimately prove your company’s commitment to security and trust, and meeting a framework can be the difference between growing your business and missing out on opportunities.
Communicate to your team that the goal is progress, not perfection. If it’s your first time getting audited against a particular framework, you probably won’t get a perfect score, and that’s okay! Instead, make it your goal to set a baseline for meeting the framework, then find ways to improve your controls over time.
Pro tip: As you and your team prepare for the audit, remember to do your due diligence before the auditor even arrives. Get to know exactly how your controls align with the framework and be realistic about which shortcomings you expect the auditor will likely find. That way, you won’t be surprised by the audit’s findings.
Manage your evidence
As your team prepares for an audit, collecting evidence of your activities is just as important as completing the activities themselves. For example, you will likely need to draft formal security policies and protocols (for example, this is one of the requirements for SOC 2). But you can’t just say, “We have the required security policies.” You’ll need to provide your auditor with a hard copy of your policies. And this is just one of the many controls they will require you to prove with documentation.
Managing evidence gets complicated quickly, considering how many documents your auditor will require. So, it’s your job to make sure that evidence is organized and ready as soon as the auditor asks for it. Here are a few tips for compiling the proper evidence to be fully prepared when the auditor arrives:
Ensure your evidence shows consistency —not just point-in-time or static documentation. You should be able to prove that you’ve maintained the proper controls throughout your audit window. A tool like Vanta that supports continuous controls monitoring can help with this.
Compile and store your documentation in a way that simplifies sharing the relevant evidence with the auditor. You want to spend as little time as possible chasing down specific documents or screenshots during the audit. Getting organized beforehand means far less stress for your team when the auditor begins their assessment. Vanta can help you stay on top of your documentation by storing all documents in a centralized location and automatically updating data with real-time changes.
Get to know your evidence inside and out. You shouldn’t be surprised by anything that your auditor finds. It helps to use a governance, risk, and compliance (GRC) automation tool to parse through the documentation and summarize findings. This way, you have a consolidated view of evidence rather than a bunch of disparate documents and files that are hard to consume at once.
Be transparent and upfront with your evidence. Don’t try to shape a specific story or alter the truth by showing more or less documents to an auditor. Honesty is always the best policy in these cases, especially considering the potential consequences of lying in an audit. Altering the truth can significantly impact the integrity of your company, or even prevent you from requesting audits in the future.
Pro tip: Be transparent without embellishing the truth when the auditor arrives. Let your evidence speak for itself. You might think this goes without saying, but the temptation to overshare or embellish can get the best of anyone when the audit actually begins.
Work closely with your auditor
Many organizations enter the auditing process with concerns about their relationship with the auditor. Fortunately, in most cases, the process will be less like a test proctored by an intimidating professor and more of a back-and-forth conversation with an industry expert who knows best how to get you to your goals.
Here’s some advice for working with your auditor and making it a better experience for both of you:
Look for specialization. Find an auditor specializing in the framework(s) you’re working toward. You can start by asking for recommendations within your industry—Vanta’s network of trusted auditors is a great place to start.
Help your auditor understand your business. You will need to guide them through the ins and outs of your organization, including where your controls reside and which insights they provide to your business. They won't know where to start assessing your controls if they don’t understand your organization or GRC processes and tools.
Treat the audit like a conversation. In most cases, your auditor will be inside your organization for a few weeks to months, and during that time, it should become a back-and-forth working relationship. The auditor will likely ask you for specific evidence over time, and you will work with them directly to provide each piece of information they need, and so on.
Maintain the relationship with your auditor afterward. They can serve as a helpful resource as you build out your GRC initiatives and continuously maintain and improve compliance over time. While auditors can’t give direct advice on how to do something, most are more than willing to help wherever they can. For example, if you plan to change your tech stack, employee policies, etc., consider asking your auditor how these changes will impact future audits before committing to the change.
Pro tip: Keep in mind that it’s common practice to switch your auditor every few years to avoid bias. But once you’ve found a firm and auditor you like, you can always ask them for recommendations and referrals to find your next auditor.
Take your audit results to the next level
An audit isn’t just a one-and-done activity—it’s the beginning of a journey toward a stronger security posture over time. To get the most out of your audit findings, prepare your team to work on the following initiatives after the audit is done:
Share your new compliance status with the right audiences. Many businesses rely use a public-facing site (like Vanta Trust Center) to display their real-time GRC efforts. Your prospective customers can then use this data to expedite security questionnaires and provide proof points to key decision-makers.
Track KPIs that show your response to the gaps that your auditor finds. The specifics of these KPIs will depend on your particular business, priorities, etc. In general, they should center around risk reduction and remediation efforts. For instance, if you have any findings, opportunities for improvement (OFI), or nonconformities listed in your report, track the response to these insights over time. How are you changing processes? What new tools have you implemented?
Consider conducting a readiness assessment between formal external audits. Businesses will typically either hire a third party or pick a team member who isn’t involved in any GRC activities to perform this type of audit. The best way to incorporate readiness assessments into your processes without causing business disruption is to use continuous monitoring to get an instant snapshot of all existing controls.
Treat your audit like a jumping-off point for security initiatives. Meeting a particular framework doesn’t necessarily equate to bulletproof security. For example, a compliance framework often doesn’t account for emerging threats. Still, consistently meeting the controls listed in a framework is a great starting point for establishing a strong security culture throughout your organization.
Foster a strong security culture. As you respond to your audit findings and implement stronger GRC controls, your team members need to understand why specific controls exist and how to play a role in meeting them. Ultimately, this is all about fostering a strong security and risk management culture throughout your organization. Consider rolling out security training and tracking KPIs such as phishing drill click-through rates and training completion rates. This will help demonstrate progress for future audits as well as improving your organization’s security posture.
Pro tip: Keep in mind that it’s common practice to switch your auditor every few years to avoid bias. But once you’ve found a firm and auditor you like, you can always ask them for recommendations and referrals to find your next auditor.
Anatomy of an audit report
Knowing what to expect when you finally get that audit report can help you set expectations for internal and external stakeholders ahead of time. Here’s what the typical audit report should contain:
- Executive summary: A high-level recap of the report’s purpose, the standard/framework in question, etc.
- Scope: An in-depth description of the scope that the audit covered. For example, was it a corporate-wide evaluation, a test on a specific application, or something else?
- Testing information: A detailed list of all tests the auditor ran, such as policy reviews, artifact evaluations, and on-site observations.
- Testing results: A report on the results of these tests, including any exceptions.
- Management responses: The management team’s explanations as to why an exception exists and how they will respond to it in the future.
- Conclusion: The auditor’s opinion on the assessment. Keep in mind that this area of the report will rank the company by maturity, not as a pass or fail.
While compliance audits can feel overwhelming because of the time and resources that they require, they offer your team the unique opportunity to start a journey toward stronger security and deeper customer trust. Plus, tasks like scheduling, managing evidence, and working with your auditor don’t have to feel so daunting if you go into your next audit with the right expectations and preparation.
Confirm which CMMC level your business needs
The first step of the CMMC program and becoming CMMC certified is confirming what CMMC level you’re on. Here are a few ways you can confirm what level your business needs.
Determine the type of data you handle. If you handle FCI, you’ll likely fall under level 1, whereas if you handle CUI, you will be under level 2 or 3. If you handle a variety of FCI and CUI information, you must be certified at the level encompassing all the information you handle.
Check your contract. If you currently have a contract, reach out to your contracting officer. They can help determine your level and the requirements to remain compliant. As you bid on future contracts, the DoD will specify the required CMMC level and assessment type for eligibility in the solicitation and resulting contract.
Perform a self-assessment. If you don’t currently have a contract, but hope to in the future, the best thing you can do is perform a self-assessment of your systems. This ensures you meet the necessary security requirements and have implemented proper controls to protect sensitive information. It will also give you an idea of what level you’re at.
Establish your FCI and CUI boundaries
Once you confirm what level of certification you need, your next step is to figure out what systems, processes, and data—referred to as assets—fall under CMMC requirements. The DoD refers to this process as establishing your boundaries for FCI and CUI.
Review boundaries and assessment scope guidelines for your CMMC level. Each level has set boundaries and assets based on the information that’s handled. Once you know your level, you can review general guidelines for assessment scope.
Identify in-scope assets. For each level, there will be set assets that are considered in scope. Depending on the certification you’re seeking, you’ll need to identify all assets that process, store, or transmit FCI or CUI data. This includes all people, technology, facilities, and external service providers. For levels 2 and 3, this also includes specialized assets, security protection assets, and contractor risk managed assets.
Document in-scope assets. Once you’ve identified all assets in scope, you’ll need to document them. Documentation includes creating an asset inventory and providing a network diagram, which will be a part of your official assessment.
Pro tip: At each level, any asset that isn’t in scope is considered out-of-scope, meaning it doesn’t need to be documented during an assessment. For more information on what assets are considered in and out of scope, the DoD has scoping guidelines for each level.
Perform a gap assessment
Once you’ve established your CUI and FCI boundaries and your organization’s current status, you’ll want to conduct a gap assessment, also known as a CMMC gap analysis, to identify areas where your organization is falling short. A good gap assessment helps reduce the stress of prepping for CMMC certification since it’ll show you any areas or processes you need to focus on to pass level assessments and get certified. To perform a gap assessment, you’ll want to:
Identify and document existing controls. Map your current security controls to the objectives outlined in the CMMC framework. This includes documenting asset treatment in a system security plan (SSP).
Identify and document controls not yet satisfied. Identify and review controls that you haven’t yet satisfied. You’ll want to pay close attention to this step as these controls need to be addressed and satisfied to pass certification during an official assessment.
Pro tip: As you complete your gap assessment, you’ll likely need to collaborate with stakeholders across the business to ensure you have up-to-date information. Improper documentation or oversight of required controls could result in your organization not meeting CMMC certification requirements.
Create and execute a plan of action and milestones
As you work through the steps of planning and preparing for CMMC certification, you’ll also need a Plan of Action and Milestones (POA&M).
Create a POA&M. A POA&M allows companies to create a roadmap for improvement with details on what to prioritize, steps for action, who’s responsible for them, resources needed, and deadlines. Any company seeking CMMC certification can complete a POA&M after their gap assessment.
Execute your POA&M. The actions and steps outlined in your POA&M should make the process easier. But, take it step by step. Attempting to remediate every action at once makes it hard to track progress. It could also result in accidentally overlooking important steps, security gaps, or unresolved issues, which can impact your official assessment.
Regularly monitor progress and gather evidence. As you begin implementing new controls, document and monitor progress. This includes validating control design and regularly reviewing your progress against milestones. You’ll also want to gather evidence surrounding them. Evidence should include steps you’ve taken to implement controls and any results from it. If controls result in new policies and procedures, document those as well.
Pro tip: Along with completing a POA&M, you should document your Supplier Performance Risk System (SPRS) score. An SPRS score ranges from -230 to +110 and helps the DoD understand how well a contractor is protecting sensitive data and government information. Determining your SPRS score can ensure you focus on the right areas when working towards CMMC certification.
Conduct assessment
If you’ve completed steps one through six, conducting the assessment should go smoothly. Ideally, you’ve addressed all security gaps and areas of improvement in your POA&M, so there shouldn’t be any surprises during the official assessment. Like most of the CMMC certification process, the type of assessment you conduct will depend on your level.
Level 1
Conduct a self-assessment against level 1 security requirements. Level 1 only requires a self-assessment.
Gather and prepare evidence. A self-assessment requires the submission of documents and evidence, such as policy and process documents, training materials, and planning documents. It also requires interviews with employees and testing to ensure processes are followed.
Ensure all level 1 security requirements are met or marked as “not applicable.” To achieve level 1, all security requirements must be met or not applicable, with results entered into the SPRS.
Level 2
Determine which assessment is required. Level 2 has two types of assessments, a self-assessment or a certification assessment. Self-assessments for level 2 are the same as level 1. For a certification assessment, a C3PAO must complete it.
Gather and prepare evidence. The documents, interviews, and testing laid out in level 1 are required for level 2 certification.
Determine if you’re eligible for a conditional or final level 2. A level 2 assessment can result in a conditional or final level 2. A conditional level 2 will require a POA&M. To achieve level 2, all security requirements must be met or not applicable.
Update your POA&M for final certification if you receive a conditional level 2. To achieve a final level 2, you must complete or close out your POA&M within 180 days.
Level 3
Achieve final level 2 certification with a C3PAO. Level 3 requires two assessments. Both must be completed to achieve level 3 certification. The first assessment is achieving final level 2 with a C3PAO.
Complete an assessment with the Defense Contract Management Agency (DCMA) DIBCAC. This assessment is based on level 3 requirements. The DCMA DIBCAC assessment can result in a conditional or final certification.
Update your POA&M for final certification if you receive a conditional level 3. To achieve final level 3, you must complete or close out your POA&M in 180 days.
Pro tip: Keep in mind that it’s common practice to switch your auditor every few years to avoid bias. But once you’ve found a firm and auditor you like, you can always ask them for recommendations and referrals to find your next auditor.
Maintain certification
After putting in the work to officially become CMMC certified, you’ll need to maintain your certification. Due to the quickly evolving nature of cybersecurity, the DoD requires CMMC-certified organizations to review their controls and affirm compliance annually. Depending on your level, assessments take place annually or every three years.
Level 1
Complete a self-assessment annually. Similar to initial certification, you must complete the self-assessment and enter it into the SPRS every year.
Complete an annual affirmation. The annual affirmation verifies compliance with the 15 basic safeguarding requirements in FAR clause 52.204-21, Basic Safeguarding of Covered Contractor Information Systems.
Level 2
Complete an annual affirmation. For both self and C3PAO certifications, an annual affirmation verifying compliance with the 110 security requirements in NIST SP 800-171 Revision 2, Protecting Controlled Unclassified Information in Nonfederal Systems and Organizations needs to be completed and entered into the SPRS.
Complete a self- or C3PAO assessment every three years. Results of a self assessment must be entered into the SPRS. C3PAO results must be entered into the CMMC Enterprise Mission Assurance Support Service (eMASS).
Level 3
Complete an affirmation annually. An annual affirmation must be completed to verify compliance with the 24 identified requirements from NIST SP 800-172 and entered into the SPRS.
Complete a DIBCAC assessment every three years. Results of a DIBCAC assessment must be entered into CMMC eMASS.
How Vanta makes CMMC certification easier
While CMMC certification can feel overwhelming, having tools to help you prepare for and maintain certification is key.
Vanta makes it easier to meet CMMC requirements across all three levels. Through a centralized platform, Vanta can guide you through the step-by-step process of obtaining and monitoring your CMMC implementation.
Additionally, Vanta’s extended partner network offers hands-on support to ensure you can successfully implement CMMC and conduct assessments to renew contracts and win new business.
A note from Vanta: Vanta is not a law firm, and this article does not constitute or contain legal advice or create an attorney-client relationship. When determining your obligations and compliance with respect to relevant laws and regulations, you should consult a licensed attorney.
Starting your healthcare compliance program
Starting a healthcare compliance program may seem complex, particularly if you’re new to security and compliance. But with the right approach, it can become a clear and manageable process. Investing in building a strong foundation now will set your organization up for future scale and success.
Evaluate your business needs: Start by assessing your organization’s compliance drivers including the following questions:
- Do you collect or process PHI? If yes, HIPAA compliance is mandatory.
- Have clients or prospects ever asked you about compliance with HIPAA or other industry security standards?
- Do your competitors maintain HIPAA compliance or other security standards such as SOC 2, ISO 27001, or HITRUST?
Confirm your PHI scope and data flows: Map out how PHI is collected, processed, transmitted, and stored. You’ll want to minimize PHI exposure across systems and vendors and implement the principle of least privilege and minimum necessary use, in alignment with both HIPAA and broader information security best practices to help reduce risk.
Engage legal counsel and define key roles: As noted above, HIPAA compliance is a legal obligation if your organization handles PHI in any way. You’ll want to review and define your role as a Covered Entity or Business Associate and involve legal counsel early in the process to help guide your regulatory compliance scope and strategy.
Get HIPAA-ready: To prepare for HIPAA compliance, start by implementing baseline technical and administrative safeguards, such as enabling automatic session timeouts and screen locks, encryption at rest and in transit, multi-factor authentication (MFA) enforcement, and least privilege access configurations. Next, establish policies covering various domains such as access control, incident response, breach notification, mobile device management, as well as patient privacy, rights, and related disclosures. From a third-party perspective, ensure you sign Business Associate Agreements (BAAs) with all third parties that handle PHI, and conduct vendor risk assessments to validate their HIPAA compliance posture.
Layer additional frameworks for audit assurance: To strengthen your healthcare compliance program and meet growing customer expectations, consider additional frameworks such as SOC 2 or ISO 27001/27017. These standards provide a more structured and auditable foundation for your compliance practices, and when paired with HIPAA, they enhance your security and privacy posture while also signaling to customers and prospects that you’re prepared to meet their expectations for trust.
Accelerate compliance with the right technology: Instead of relying on manual processes and spreadsheets, choose a platform that streamlines and automates your path to audit readiness—especially if you're working with a lean team. To accelerate sales conversations, build an external-facing trust center to proactively showcase your security posture and healthcare compliance to prospects. You can enhance your trust center by automating security questionnaires with AI-powered responses.
Pro tip: Vanta simplifies your healthcare compliance journey by outlining exactly what’s needed to get audit-ready. Vanta identifies gaps, shows you how to fix them, and automates the process.
Scaling your healthcare program
As your business grows, your compliance program needs to scale with it. Whether you're expanding your team, adding new systems, or selling to larger customers, complexity increases—and so does the need for a more mature and efficient approach to security and compliance. This phase is about operationalizing your program with the right frameworks, tools, and processes to stay ahead of risk while maintaining velocity.
Identify efficiency gaps: Growth introduces new challenges: more people, more tools, and more moving parts. As your business scales, your current compliance processes may not be able to keep up with the additional complexity and operational overhead. Assess your maturity needs by asking the following questions:
- Is your company expanding rapidly or pursuing enterprise clients?
- Are you adding more tools or systems to your environment?
- Is it becoming harder to track compliance status across teams or frameworks?
If you answered yes to any of these questions, then it's time to shift from a foundational program to a scalable and more integrated model.
Mature your program with more prescriptive frameworks: While HIPAA is a necessary baseline, it’s not designed to provide the depth of guidance needed for large-scale or highly regulated operations. As expectations increase, consider layering in additional frameworks that provide further structure, auditability, and credibility.
- HITRUST: Offers tiered, prescriptive certification paths (e1, i1, r2) tailored to healthcare use cases and often required by enterprise customers, such as large health providers.
- NIST CSF: Helps align with cybersecurity risk management best practices, especially if you plan to work in the public sector.
- NIST AI RMF or ISO 42001: If you're incorporating AI, these frameworks support responsible AI governance and risk management.
Centralize and automate compliance workflows: As complexity grows, fragmented workflows and manual tracking break down. Implementing a trust management platform can help you reduce operational overhead and improve visibility by:
- Enabling cross-mapping of controls, so you only have to test once across multiple frameworks, such as between HIPAA, SOC 2, HITRUST, ISO 27001, etc.
- Running automated tests to continuously monitor your controls for failure risks and streamline year-round audit readiness.
- Sending alerts when gaps are identified, and providing clear remediation steps.
Strengthen vendor risk management: Your risk surface grows with every third-party vendor you add. Taking a proactive approach to vendor management not only protects your sensitive data, such as PHI, but also signals maturity to your customers and partners. This includes:
- Building a centralized inventory of all vendors that handle PHI or your sensitive and critical systems.
- Tracking BAAs, SOC 2 reports, and other security and privacy documentation
- Automating vendor risk reviews and renewal cycles to ensure continuous oversight.
Stay ahead of emerging regulation: The healthcare regulatory environment continues to evolve, especially with the rise of AI and ongoing threats to PHI. To stay compliant over time, keep an eye on:
- Updates to regulations, such as HIPAA. Examples include the upcoming Security Rule update.
- New laws such as Healthcare Cybersecurity Act, which may introduce new federal expectations for providers and vendors.
Additionally, build time into your roadmap for regular gap assessments and policy reviews. Staying proactive helps you reduce risk, avoid fire drills, and respond to change with confidence.
Build trust and drive growth with Vanta
Vanta automates HIPAA, HITRUST, SOC 2, and 35+ other frameworks to help you simplify compliance, strengthen security, and boost efficiency—all in one place. Reduce manual work and monitor compliance continuously, reducing the hassle of screenshots and spreadsheets. And as your business grows, Vanta scales with you, helping you save time on evidence collection with 375+ integrations and automatic cross-mapping between frameworks.
Learn more about Vanta for Healthcare.
A note from Vanta: Vanta is not a law firm, and this article does not constitute or contain legal advice or create an attorney-client relationship. When determining your obligations and compliance with respect to relevant laws and regulations, you should consult a licensed attorney.
Understand your risk level
The first step to becoming AI Act compliant is to understand your risk level. The AI Act specifies four categories to determine this:
Unacceptable: This class of AI is considered a clear threat to those who use it, with harmful features aimed at manipulation and exploitation. It is prohibited as a result.
High: High-risk AI poses serious safety and health risks, and potentially interferes with people’s fundamental rights. However, these cases can reach the market if they meet strict security obligations.
Limited: This category covers AI with specific transparency requirements, e.g. chatbots that must inform users that they are interacting with AI.
Minimal (no risk): This AI poses minimal or no risk to people with the majority of AI use cases across Europe falling into this category.
Understanding your risk level isn’t just about your organisation though – it’s about those you work with. You should therefore extend your AI risk categorisation to the third-party vendors you work with.
Understand the AI risk level of your third-party vendors and the AI they’re using.
Understand the AI risk level AI of your AI-driven vendors, such as LLM providers
Meet regulation requirements and establish AI risk management
The next step is to work on the AI Act regulation requirements as per your risk level to ensure you are compliant. You must ensure this covers best practices around:
- User research
- System architecture related to your AI system
- Data collection and model training to insure integrity and quality of your AI systems are maintained
- Documentation to be able to explain your AI risk management system
- Feedback mechanisms
Comply with the NIST AI RMF: Regardless of your risk level, you should establish and maintain an AI risk management system–one that includes mitigation strategies. An effective method of doing this is to follow the NIST AI Risk Management Framework. As part of this, you should leverage technical measures for risk mitigation and human-performed activities such as risk management software, automation and even AI itself. But you should also establish human oversight mechanisms for risk management to ensure you are bringing human oversight and accountability.
Obtain the right technical documentation
It is important to obtain the right documentation to support compliance assessment. A good place to start is with an Information Security Management System (ISMS). This establishes a systematic approach to managing your organization’s information security, and a structured approach to integrating information security into your business processes.
Helping to manage and minimise risks to acceptable levels will increase your organization’s resiliency against evolving security threats and ensures the confidentiality, integrity, and availability of organizational and customer information. The scope of an organization’s ISMS can be as small or as large as is necessary. The ISO 27001 standard defines which documents must exist at a minimum.
Plan your ISMS: Put a team and a roadmap together to implement ISMS policies and controls, and continuously assess risk.
Meet the ISO 27001 standard: ISO 27001 is a go-to standard that aligns with global and EU-specific data protection regulations like the GDPR. You can find our ISO 27001 Compliance Checklist here.
To become AI Act compliant, you should establish an Artificial Intelligence Management System (AIMS). ISO 42001 is a great place to start as it is the international standard for establishing, implementing and maintaining an effective AIMS:
Plan your AIMS: Assign a project leader and put a team and roadmap together to implement your AIMS. Our ISO 42001 checklist is a great place to kick this off in the right way.
Earn ISO 42001 certification: ISO 42001 certification demonstrates to customers, partners and regulators that you’re using AI responsibly, ethically and transparently.
To truly demonstrate trust, companies should go beyond the standard. For fintechs, and other financial institutions, this might look like compliance with the Digital Operational Resilience Act (DORA) framework.
Comply with the DORA framework: Not strictly part of the AI Act, the DORA is a relatively new EU framework for ensuring enhanced cybersecurity and operational resilience of financial entities operating in the Union.
Pro tip: You can find out more about DORA and how Vanta can help fintechs scale trust in our free guide–Fortifying Fintech: Security must-haves for Europe’s trailblazers.
Establish data governance
Data governance is essential to maintaining trust and companies must ensure the confidentiality, availability and integrity of the information they handle.
The data used by AI is not just about quality, however–it is about the transparency surrounding how you use it. More and more organisations are training AI models on a mix of customer and synthetic data. What’s more, without a formal data processing agreement (DPA) that prohibits vendors from using customer data to train their AI models, companies risk threats coming from third-parties too.
Ensure you have a formal DPA with your third-party vendors.
Ensure the quality of your data for training, validation, and testing of AI systems.
Ensure your data is accurate, representative and complete.
Ensure customers are given an opt-out option for training AI with their customer data.
Build with trust and transparency
In the AI era, trust and transparency are essential. Customers must have easy access to appropriate information about your company and products, e.g. characteristics, capabilities, limitations, potential risks.
This can be an all-consuming process for security teams, which is why we launched Trust Center – a hassle-free way for companies to demonstrate trust in real-time. Because earning security credentials is only half of the job–to demonstrate trust, you have to communicate them too.
Set up a process to collect evidence to demonstrate compliance: Demonstrating compliance can come with a lot of chaos. But Vanta can help you to automatically gather the evidence you need – taking the strain off your security team.
Communicate security credentials: Use Vanta’s Trust Center to communicate your security credentials to customers, demonstrate trust and turn good security into good business.
Embrace continuous compliance
Forward-thinking organisations won’t stop at the AI Act. They’ll go beyond point-in-time checks towards a holistic and continuous approach to monitoring. This is the practice of ensuring that you’re properly meeting compliance standards you’ve committed to on an ongoing basis – not just at the time of an audit. This approach is integral to establishing appropriate levels of cybersecurity, accuracy and robustness.
Embrace continuous compliance: The path to continuously monitoring your systems looks like checking for compliance gaps on a daily basis – and relying on automation to cut the amount of manual work this involves.
Continuous compliance will ensure you are in a good position to monitor for updates from the European Commission and that you remain agile to the broader risk landscape. But it will also bring reputational benefits and a welcome boost to your bottom line.
Streamline your EU AI Act compliance with Vanta
Vanta is the fastest way to become AI Act compliant, strengthen your cybersecurity posture, and build trust. Vanta’s trust management platform provides organisations with the capabilities and guidance required to efficiently and effectively achieve AI Act compliance. It provides a centralised view of your compliance and security posture by continuously monitoring the critical tools and services your business runs on. Get in touch with our team today.
Pre-work for your Cyber Essentials certification
Evaluate your need for Cyber Essentials. You can assess whether you need a Cyber Essentials certification based on the following criteria:
Do you collect or process sensitive or personal data from UK residents?
Have you ever been asked about Cyber Essentials by clients or prospects?
Do your competitors have Cyber Essentials certification?
Are you working with companies that provide public services in the UK?
Choose the right Cyber Essentials certification. Although both versions of the Cyber Essentials frameworks have the same requirements, Cyber Essentials Plus involves a third-party audit and technical testing. As a result, Cyber Essentials Plus provides an extra layer of security validation beyond the self-assessment in the standard Cyber Essentials certification.
Do you need Cyber Essentials or Cyber Essentials Plus?
Here are some helpful considerations for deciding whether to pursue the Cyber Essentials certification or Cyber Essentials Plus:
Determine your budget. Cyber Essentials Plus is more expensive than Cyber Essentials because of the external audit and higher security assurance. Pricing for Cyber Essentials ranges from £320 to £600 (plus VAT). Cyber Essentials Plus does not have a fixed pricing structure, but estimates start at £1,499 (plus VAT) and increase based on your organisation’s size and complexity. Learn more about Cyber Essentials costs.
Assess your time and team resources. Cyber Essentials Plus requires more time and bandwidth and takes longer to complete, especially for smaller companies (or security teams) with limited internal resources.
Understand your stakeholders’ concerns. The Cyber Essentials Plus third-party audit requirement enables you to demonstrate your commitment to enterprise-wide data security and assure stakeholders who may have security concerns.
Define customer requirements. Cyber Essentials Plus is required to win UK government contracts. It may also be required to conduct business with certain companies in the UK, such as those looking to prevent potential supply chain-related cyber threats from impacting their operations.
Evaluate your infrastructure size and complexity. Larger, more complex entities whose interconnected assets and operations are at high risk of being disrupted by cyber threats may benefit from Cyber Essentials Plus. Its rigour ensures reliable safeguards protect this infrastructure.
Estimate the volume and sensitivity of stored data. Companies that handle vast amounts of sensitive data (such as PHI in healthcare) may gain higher assurance with Cyber Essentials Plus.
Prepare for your Cyber Essentials certification
First, identify your Cyber Essentials requirements.
Obtaining a Cyber Essentials certification requires you to implement controls across five categories:
Firewalls: All firewalls deployed to system boundaries, endpoints, servers, cloud services, and other critical infrastructure entry points should have the correct security configurations, access controls, and security rules.
Secure configurations: All configurations applied to endpoints and networked services should reduce exploitable vulnerabilities while enabling the completion of business-critical services.
User access controls: Access to all user accounts should require authorisation, and only the people who need access to specific endpoints, services, or applications to complete business tasks should have access. Consider using time-bound or just-in-time access and implementing the principle of least privilege (PoLP) to tighten user access controls.
Malware protection: Administrators should establish the appropriate security mechanisms to prevent malware intrusion via known and unknown sources. They should also educate employees and third parties on the risks of malware and ransomware and how to avoid social engineering scams.
Security updates: All devices and software at risk for security flaws should be updated regularly to ensure their configurations are secure and in alignment with recommended industry/manufacturer security standards.
Gap analysis and remediation
Conduct a gap analysis. Evaluate IT infrastructure for gaps in the above control categories.
Document gaps and develop a remediation plan. Documenting the gaps identified and proposing actionable steps to rectify them is critical.
Address gaps that could lead to security vulnerabilities. Following a gap analysis, you may need to remediate sources of exploitable vulnerabilities.
Examples of remediation steps include:
Changing default administrative passwords into stronger, unique ones
Deactivating unnecessary user accounts that are not currently in use
Scheduling automatic system and network security updates
Implementing multi-factor authentication for all user accounts
Installing anti-malware software on all devices
Test all applicable controls. It’s critical to monitor devices and networks to verify the effectiveness of implemented controls in preparation for assessment. Test your controls and make sure they are functioning properly.
Document findings from system monitoring. It’s also helpful to record all the information from monitoring in an organised, shareable report format to keep track of it. Doing so manually is a challenge for any organisation, which is where automation solutions, like Vanta, help reduce these burdens and enable organisations to scale their security monitoring capabilities as they grow.
Complete the self-assessment (for Cyber Essentials)
After you’ve identified the Cyber Essentials requirements that apply to your organisation and remediated any security gaps, the next step towards becoming Cyber Essentials certified is to complete the self-assessment, which is required even when you’re aiming for the Cyber Essentials Plus certification. Here are the steps our team of compliance experts recommend taking:
Prepare for the Cyber Essentials self-assessment. Train all parties involved in the certification process so they are on the same page regarding Cyber Essentials documentation. Building and maintaining a security awareness culture is crucial for your organisation to fully comply with Cyber Essential security guidelines during its day-to-day operations beyond preparing for the Cyber Essentials self-assessment (or audit, if you choose to pursue Cyber Essentials Plus).
Conduct the self-assessment questionnaire. (SAQ). When ready, you can complete an online SAQ and have a senior team member validate it to get the Cyber Essentials certificate. It’s essential to be accurate and truthful when answering questions to ensure alignment with the necessary standards for obtaining the certificate.
Use online readiness tools to prepare for the test. Tools like the IASME Cyber Essentials Readiness Tool provide free questions to help you assess the posture of your company’s controls and their impact on your business operations. These questions are designed to inform you about your current readiness so you can develop a tailored action plan for the Cyber Essentials audit.
Decide whether you need the Cyber Essentials Plus audit. You can choose to obtain the Cyber Essentials Plus certification immediately after the Cyber Essentials self-assessment or in the following months. While you must complete just the self-assessment to obtain the Cyber Essentials certification, you’ll also need to complete and pass an external audit to receive the Cyber Essentials Plus certification.
Complete the external audit for Cyber Essentials Plus
Prepare for the Cyber Essentials Plus audit. If you want to obtain a Cyber Essentials Plus certification, you should ensure your SAQ was certified within three months of applying for the Cyber Essentials Plus.
Identify an independent assessor: Work with a highly-trained assessor to verify that all currently implemented controls align with the Cyber Essentials Plus requirements.
Review audit findings and implement remedial actions (if any): Address any gaps identified during the independent assessment within a set period.
Obtain Cyber Essentials Plus certification: A successful Cyber Essentials Plus certification provides a certificate valid for 12 months following the assessment pass date. Then, you can become designated as a CE-certified company, opening new business opportunities in the private sector or with the UK government.
Maintain ongoing Cyber Essentials or Cyber Essentials Plus certification
Monitor your IT infrastructure regularly. Organisations need continuous internal assessments to verify adherence to the Cyber Essentials security guidelines. As systems and networks age, they are exposed to more vulnerabilities. So, you need to review your existing security configurations and ensure they still match the rigorous standards of the Cyber Essentials or Cyber Essentials Plus program.
Renew your Cyber Essentials certification.The Cyber Essentials and Cyber Essentials Plus certifications expire after 12 months and must be renewed yearly. Renewing these certifications helps you maintain your designation as a Cyber Essentials-certified company and provides assurance to current and future stakeholders.
Implement zero-trust principles. As the cyber risk environment becomes more complex, consider implementing zero trust across systems and networks to keep your data safe. New technologies are constantly emerging, meaning cybercriminals are also looking for the easiest gaps to exploit to access sensitive data for malicious gain.
Streamline Cyber Essentials certification with Vanta
A strong security posture will enable organisations to build customer trust and expand business to or within the UK. However, it’s cumbersome to manually keep track of all the processes necessary to obtain Cyber Essentials or Cyber Essentials Plus certification.
Fortunately, Vanta automates up to 70% of the UK Cyber Essentials process (including evidence collection), dramatically simplifying the compliance process. This enables teams to focus on strengthening their security programs without redundancy.
Plus, with Vanta, you don’t need to duplicate your compliance efforts across multiple frameworks. UK Cyber Essentials has significant overlap with frameworks like ISO 27001. If you’re already compliant with ISO 27001, you don’t need to reinvent the wheel; Vanta automatically cross-references other frameworks and surfaces any overlapping controls that you already have in place.
Vanta’s trust management expertise can help startups and early-stage companies in the UK build and optimise their security programs to obtain the Cyber Essentials certification. Thousands of global customers rely on Vanta’s real-time, transparent trust management platform to demonstrate their focus on security and privacy to stakeholders.
Learn more about how Vanta automates Cyber Essentials compliance.
Prework
Determine if CPS 234 is right for your organisation. If your organisation operates in Australia and is in an APRA-regulated industry or works with APRA-regulated entities, you’ll need to get CPS 234 compliant. If you aren’t required to be CPS 234 compliant, consider whether it’s beneficial for your business to align with the standard based on your customers and industry.
Take the time to understand the requirements and regulations. CPS 234 is a comprehensive compliance framework. Organisations need to follow and meet stringent information security practices to comply. While this checklist will take you through everything you need to know, understanding what CPS 234 means before you start can help you navigate the process.
Address gaps that could lead to security vulnerabilities. Compliance with CPS 234 includes involving key stakeholders and educating them on their part in the process. In many cases, each role is responsible for the steps in this checklist, including identifying assets and controls, implementing additional controls, and maintaining compliance with the framework. Positions that should be involved in the process are:
Board of Directors: Holds ultimate responsibility for the entity's information security. The board has to ensure the company maintains strict information security measures.
Senior Management: Oversees the implementation and maintenance of information security measures.
Information Security Personnel: Individuals tasked with the operational aspects of information security, including monitoring, incident response, and compliance.
Identify assets and assess controls
Identify and inventory all assets. As you start to implement CPS 234, you’ll need to identify and inventory all of your information assets. Along with your organisation’s assets, you’ll also need to identify and inventory the assets of any third-party vendors. This includes, but is not limited to:
Customer data
Financial records
IT equipment, like computers, servers, and network devices
Cloud systems
Data hosted by cloud providers
Information hosted by third-party vendors
Classify all assets. Once assets are identified and inventoried, they must be classified based on their importance, including their criticality and sensitivity. This includes how likely a security incident involving this asset could impact the business or customer. When reviewing and classifying assets, confirm whether noncritical or nonsensitive assets could impact your critical and sensitive assets. If so, they should be included.
Assess controls. Identifying and assessing your security controls means making sure they can effectively protect your sensitive and critical information assets. In this step, you’ll want to ask several questions to make sure you have the right controls, like:
Are there any current or potential threats that could impact your systems or information security assets?
Do the most critical and sensitive assets have more robust controls than less critical and sensitive assets?
What lifecycle stage is the information asset in? Is it new, used daily, or no longer in use?
How would a security incident or cyberattack impact your assets? Would any be compromised?
Do third-party vendors or other companies you work with secure your data with robust security controls?
Pro tip: Consider performing a mapping exercise to align CPS 234 controls with other standards, like ISO 27001 and SOC 2, if your organisation maintains multiple frameworks.
Implement controls. If you identify a gap or weakness in security controls, you’ll want to implement more robust standards in this step. After documenting, assessing, and prioritizing risks associated with the weakness, you’ll create a plan to fix it. Once the fix and security controls are implemented, you’ll complete testing to verify the fix and document every step you took for any future audits or reviews.
Pro tip: Consider performing a mapping exercise to align CPS 234 controls with other standards, like ISO 27001 and SOC 2, if your organisation maintains multiple frameworks.
Manage third-party risks and incident response
Properly manage all third-party risks. A key part of information security is that third-party risks are consistently managed and maintained. Organisations should confirm that third parties comply with information security standards when processing your data and accessing assets. Third parties should also have security control measures in place to mitigate risk related to security compliance.
Have a strong incident response plan. Your organisation should have a comprehensive incident response plan. The plan should cover how your organisation detects and responds to information security incidents. The plan should also include key stakeholders' roles and responsibilities during an incident. Incident response plans must be reviewed annually to make sure they are effective.
Monitor, test, and maintain compliance
Monitor and audit controls. For a comprehensive view of your organisation’s security posture, controls should be continuously monitored for effectiveness through internal audits, including controls managed by third parties. Monitoring and auditing controls can prevent unknown vulnerabilities or gaps that could impact assets.
Implement a systematic testing program. To comply with CPS 234, organisations need to implement a systematic testing program to assess the security of its controls. The frequency and type of testing should align with emerging and existing vulnerabilities and threats, the criticality and sensitivity of information assets, and the potential consequences of an information security incident.
Report to the Board. The Board must be kept informed of material information security matters. Establish a reporting mechanism to ensure the Board is regularly informed of the organisation’s information security posture, incidents, and improvements.
Continue to follow APRA regulations. If there is a security incident, organisations must notify APRA as soon as possible, but no later than 72 hours after becoming aware of a material security incident, to remain compliant. Additionally, they must inform APRA within 10 business days if they identify a material information security control weakness that cannot be remedied promptly.
Pro tip: It’s essential that APRA-regulated businesses maintain compliance with CPS 234. Noncompliance can result in hefty fines and operational and business restrictions, including direct legal consequences for senior executives.
How Vanta can help with CPS 234 implementation
Whether you’re becoming CPS 234 compliant or looking for an easier way to maintain it, Vanta can help. Vanta automates the prep work for CPS 234 compliance by centralizing requirements and guidance in one tool. Vanta also provides continuous monitoring, providing an additional layer of security to build customer trust.
Vanta can make your journey to CPS 234 compliance easier, helping your team scale faster while maintaining safety and security.
Talk to us today.
A note from Vanta: Vanta is not a law firm, and this article does not constitute or contain legal advice or create an attorney-client relationship. When determining your obligations and compliance with respect to relevant laws and regulations, you should consult a licensed attorney.
Pre-work for your HITRUST certification
Align your goals: Ensure that pursuing a HITRUST certification aligns with your business goals and programs
Find internal stakeholders: Identify key stakeholders within your organization, such as champions who can drive internal buy-in, and a project owner to drive the certification process
Educate leadership: Educate your team, executives, and the board on the HITRUST process, implied changes, and the added value to your organization of attaining certification
Work for your HITRUST certification
Understand the HITRUST CSF: Familiarize yourself with the control requirements of the HITRUST CSF
Identify compliance needs: Determine your organization's specific compliance requirements
Select appropriate HITRUST assessment type: Choose between e1, i1, or r2 assessment, according to needs of your business and your risk profile
Use Vanta to streamline the assessment: Use Vanta to identify and remediate gaps in your compliance program
Find an external assessor: Work with Vanta to select a HITRUST Validated Assessor—A-LIGN, Prescient Assurance, or Insight Assurance—to guide you through and to conduct your Readiness assessment
Purchase MyCSF subscription: Work with Vanta to initiate the procurement process for your Validated Assessor, MyCSF subscription, and report credit (if required)
Attend the HITRUST course: Gain insights and guidance through HITRUST’s New Customer Orientation
Define assessment scope: Identify the areas and systems to be included in the assessment and communicate the intended timeline of certification to your chosen Validated Assessor
Perform initial assessment: Work with your external assessor to identify gaps using Vanta
Initiate inheritance plans: Create plans for internal or external inheritance of controls
Make inheritance requests: Submit inheritance requests via the MyCSF platform
Secure QA date: Schedule a date for post-submission HITRUST QA review
Remediation
Resolve gaps: Use Vanta to identify and remediate any evidence gaps identified during the readiness assessment
The Validated Assessment
Provide evidence: Collect and submit necessary evidence to your external assessor
Finalize inheritance: Confirm all inheritance plans
Support External Assessor: Be available for any additional validation requests
Address issues: Resolve any issues noted during the pre-submission QA
Submission and Review
Submit assessment: Submit the relevant evidence to your chosen Validated Assessor to input into MyCSF for HITRUST to review
Support QA process: Be available to assist your external assessor with any QA queries
Certification
Review certification report: Check and approve the draft certification report
Ensuring compliance over time and beyond
Ongoing Monitoring
Remediate gaps: Use Vanta to continue to monitor your state of compliance and continue to close gaps
Plan for the next assessment
Future planning: Prepare for your next HITRUST assessment to maintain compliance, manage risks, and enhance security —
leveraging inheritance where appropriate
Advancing your assessment: Consider expanding your security posture by exploring the different levels within the HITRUSTecosystem — from e1 to i1 and i1 to r2
Leverage the business benefits of HITRUST certification, including
Commercial compliance: Satisfy customer, contractual requirements or preferences for HITRUST certification
Market access: Gain access to new or additional markets that require or prefer HITRUST certification
Market differentiator: Demonstrate the highest level of security posture as a vendor or partner
Risk mitigation: Adopt proven, repeatable, and measurable methods ensure significant risk reduction in certified environments
(only 0.64% of experienced breaches over two years)
Value creation: Improve potential valuation with investors and shareholders
Liability reduction: Protect yourself and your organization by using a prescriptive, complete, and proven framework to plan,
build, execute, and validate your cybersecurity program instead of building your own
Regulatory compliance: Reuse and restate HITRUST validated controls using HIPAA, SOC 2, ISO 27001, GDRP, and others to reduce compliance efforts
Download this checklist for easy reference
Download NowScoping and assessment planning
Determine business requirements
Identify which customers or partners require HITRUST certification
Document specific contractual obligations related to HITRUST
Clarify which assessment level (e1, i1, or r2) is needed to meet requirements
Define certification scope
Identify systems and applications that store, process, or transmit sensitive data
Document data flows within and outside the organization
Determine which business units and facilities are in scope
Map organizational structure and roles involved in maintaining controls
Build your HITRUST roadmap
Create a 24-36 month progression plan (typically e1 → r2)
Set realistic timeline milestones based on organizational readiness
Allocate budget for assessment, tools, and potential remediation
Identify resources needed for each phase of certification
Governance and resource alignment
Establish executive sponsorship
Secure leadership buy-in for the HITRUST certification initiative
Define executive roles in oversight and governance
Establish a reporting structure for compliance progress
Assemble your HITRUST team
Designate a HITRUST program manager
Identify control owners across departments (IT, HR, Legal, etc.)
Consider hiring or contracting specialized HITRUST expertise
Establish regular coordination meetings for the compliance team
Select implementation partners
Evaluate HITRUST-experienced consultants (like Workstreet)
Choose your HITRUST Assessor Organization
Implement a compliance management platform (like Vanta)
Gap assessment and remediation planning
Perform initial gap analysis
Assess current policies against HITRUST policy requirements
Evaluate existing procedures against HITRUST's prescriptive requirements
Identify gaps in documentation and evidence collection
Document technical control deficiencies
Develop remediation strategy
Prioritize gaps based on risk and implementation complexity
Create detailed remediation plans with owners and deadlines
Establish tracking mechanism for remediation progress
Allocate resources for policy development and technical remediation
Address HITRUST's most common challenge areas
Ensure policies and procedures are prescriptive and align with implementation
Create process to maintain evidence of security awareness training
Establish robust vulnerability management program with clear timelines
Implement comprehensive access control and review processes
Develop detailed business continuity and disaster recovery plans
Implementation and evidence collection
Develop and revise documentation
Revise policies to meet HITRUST's prescriptive requirements
Ensure procedures are detailed enough to guide actual implementation
Develop supporting materials (training, forms, templates)
Establish access control and approval processes
Implement technical controls
Address identified technical gaps from assessment
Leverage Vanta to validate technical implementations meet HITRUST requirements
Document configuration settings and technical specifications
Perform testing to ensure controls operate as intended
Establish evidence collection process
Set up automated evidence collection through Vanta
Create calendar for manual evidence collection requirements
Implement evidence storage and organization system
Document evidence collection procedures for control owners
Pre-assessment validation
Conduct internal readiness assessment
Perform mock assessment of all controls
Validate evidence completeness and quality
Test understanding of control owners who may be interviewed
Review scoring potential based on HITRUST's strict scoring criteria
Refine implementation based on findings
Address any identified weaknesses from internal assessment
Strengthen documentation where needed
Collect additional evidence for problematic controls
Conduct follow-up validation on remediated areas
Remediating and testing controls
Run tests on new and existing controls
Prepare for assessor engagement
Organize evidence package for assessor review
Brief control owners on assessment process and expectations
Schedule key stakeholders for availability during assessment
Create communication plan for assessment period
Working with Vanta and Workstreet breaks down barriers to building and operating a successful HITRUST program.
As Vanta's largest services partner, Workstreet brings specialized knowledge to your HITRUST journey:
- Strategic planning: Build a realistic roadmap from e1 to r2 certification over a 24-36 month timeline
- Policy development: Create the prescriptive documentation HITRUST demands without starting from scratch
- Implementation guidance: Navigate the strict scoring requirements that catch many organizations by surprise
- Assessment management: Coordinate with assessors and prepare your team for interviews and evidence reviews
- Remediation support: Address gaps efficiently with proven approaches from our extensive experience
See how Workstreet can take you from zero to compliant on Vanta in under 30 days.
Determine which annual audits and assessments are required for your company
Perform a readiness assessment and evaluate your security against HIPAA requirements
Review the U.S. Dept of Health and Human Services Office for Civil Rights Audit Protocol
Conduct required HIPAA compliance audits and assessments
Perform and document ongoing technical and non-technical evaluations, internally or in partnership with a third-party security and compliance team like Vanta
Document your plans and put them into action
Document every step of building, implementing, and assessing your compliance program
Vanta’s automated compliance reporting can streamline planning and documentation
Appoint a security and compliance point person in your company
Designate an employee as your HIPAA Compliance Officer
Schedule annual HIPAA training for all employees
Distribute HIPAA policies and procedures and ensure staff read and attest to their review
Document employee trainings and other compliance activities
Thoroughly document employee training processes, activities, and attestations
Establish and communicate clear breach report processes
to all employees
Ensure that staff understand what constitutes a HIPAA breach, and how to report a breach
Implement systems to track security incidents, and to document and report all breaches
Institute an annual review process
Annually assess compliance activities against theHIPAA Rules and updates to HIPAA
Continuously assess and manage risk
Build a year-round risk management program and integrate continuous monitoring
Understand the ins and outs of HIPAA compliance— and the costs of noncompliance
Download this checklist for easy reference
Download Now
FEATURED VANTA RESOURCE
The ultimate guide to scaling your compliance program
Learn how to scale, manage, and optimize alongside your business goals.